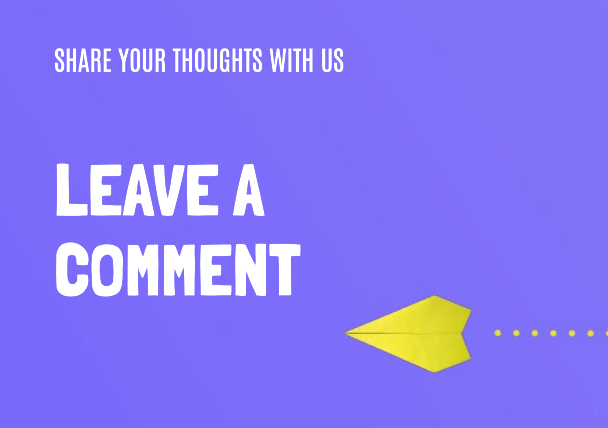
HTML for Structuring Comments
Before we dive into creating a comment section, it’s essential to understand how HTML structures content. HTML, which stands for HyperText Markup Language, is used to structure content on the web. In the context of a comment section, we’ll focus on using HTML to structure the layout.
1. Create the Basic Structure
Start by creating the basic structure of your webpage using HTML. Typically, this involves using HTML tags such as <html>
, <head>
, and <body>
. Here’s a minimal example:
<!DOCTYPE html>
<html>
<head>
<title>Comment Section Example</title>
</head>
<body>
<!-- Your content goes here -->
</body>
</html>
2. Add a Title
Inside the <head>
section, add a title for your webpage using the <title>
tag. This title will be displayed in the browser’s title bar.
3. Create a Section for Comments
Within the <body>
section, create a container for your comments. You can use various HTML elements to structure this section, such as <div>
, <section>
, or <article>
. For example:
<section id="comment-section">
<!-- Comments will be displayed here -->
</section>
HTML for Displaying Comments
To display comments on your webpage, you’ll need to use HTML and potentially some CSS for styling. Here’s how you can structure and display comments:
1. Create Individual Comment Elements
Each comment should be structured as an individual element within your comment section. You can use HTML elements like <div>
, <article>
, or <li>
to represent each comment. For instance:
<div class="comment">
<h3>User Name</h3>
<p>This is a comment...</p>
</div>
2. Style Your Comments
To make your comments visually appealing, you can use CSS to style them. CSS allows you to control the colors, fonts, spacing, and overall layout of your comments. Here’s an example of CSS code to style comments:
.comment {
border: 1px solid #ccc;
padding: 10px;
margin: 10px;
background-color: #f9f9f9;
}
.comment h3 {
font-size: 16px;
margin: 0;
}
.comment p {
font-size: 14px;
}
You can include this CSS code within a <style>
tag in the <head>
section of your HTML document or link to an external CSS file.
3. Displaying Comments Dynamically
For a fully functional comment section, you’ll need to use JavaScript or a server-side language like PHP to handle user input and display comments dynamically. This involves storing and retrieving comments from a database and updating the comment section without requiring a full page refresh.
HTML for Comment Forms
Allowing users to submit comments requires the creation of an HTML form. Here’s how you can create a basic comment form:
1. Create a Form Element
Within your comment section, add an HTML form element to collect user input:
<form id="comment-form">
<label for="username">Name:</label>
<input type="text" id="username" name="username" required>
<label for="comment">Comment:</label>
<textarea id="comment" name="comment" required></textarea>
<button type="submit">Submit Comment</button>
</form>
2. Handle Form Submission
To handle form submission, you’ll need JavaScript or a server-side script. JavaScript can be used to validate user input and send comments to a server for storage and retrieval.
Conclusion
Creating a comment section in HTML involves structuring your webpage, displaying comments, and adding a comment submission form. To make your comment section fully functional and dynamic, you’ll likely need to incorporate JavaScript and possibly server-side scripting. By following these steps, you can engage your website’s visitors and foster valuable discussions.