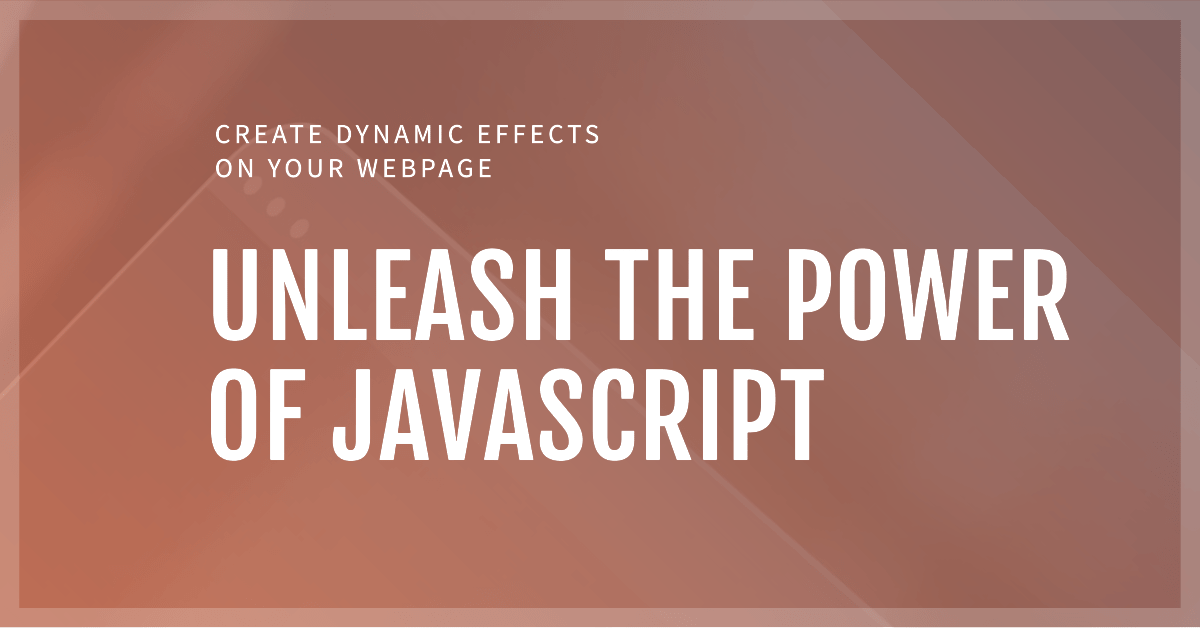
Table of Contents
In the world of web development, creating dynamic and interactive web pages is a key goal. JavaScript, a versatile and powerful scripting language, is at the heart of achieving this goal. With JavaScript, web developers can breathe life into static HTML documents, enabling them to respond to user actions, manipulate content, and create immersive user experiences. In this comprehensive guide, we’ll explore how JavaScript can be used to interact with HTML elements and craft captivating dynamic effects on a webpage.
The JavaScript-HTML Connection
JavaScript and HTML are inseparable partners in web development. While HTML structures the content and layout of a webpage, JavaScript adds interactivity and dynamism to it. The primary method for JavaScript to interact with HTML is through the Document Object Model (DOM).
What Is the DOM?
The DOM is a representation of the web page’s structure, created by the browser when it loads an HTML document. It forms a tree-like structure where each element in the HTML document is a node in the tree. JavaScript can access, manipulate, and modify these nodes, allowing for dynamic changes to the webpage.
Accessing HTML Elements
JavaScript provides several methods for accessing HTML elements, each serving a specific purpose:
1. getElementById()
This method retrieves an HTML element based on its unique ID attribute. For example, if you have an element with the ID “myElement,” you can access it like this:
let element = document.getElementById("myElement");
2. getElementsByClassName()
You can select elements based on their class names. This method returns an array-like HTMLCollection containing all matching elements.
let elements = document.getElementsByClassName("myClass");
3. getElementsByTagName()
This method selects elements based on their HTML tag name. It also returns an HTMLCollection.
let elements = document.getElementsByTagName("p");
4. querySelector() and querySelectorAll()
These methods allow you to use CSS-style selectors to access elements. querySelector()
returns the first matching element, while querySelectorAll()
returns all matching elements.
let element = document.querySelector("#myElement");
let elements = document.querySelectorAll(".myClass");
5. Accessing Elements by Their Attributes
You can also select elements by their attributes, such as data attributes or custom attributes.
let element = document.querySelector("[data-custom='value']");
Modifying HTML Elements
JavaScript empowers developers to modify HTML elements in various ways, enabling dynamic effects and interactivity.
1. Changing Content
You can change the text or HTML content of an element using the textContent
or innerHTML
properties.
let element = document.getElementById("myElement");
element.textContent = "New text content";
element.innerHTML = "<strong>New HTML content</strong>";
2. Modifying CSS Styles
JavaScript allows you to manipulate CSS styles, making it possible to change an element’s appearance dynamically.
let element = document.getElementById("myElement");
element.style.color = "red";
element.style.fontSize = "20px";
3. Adding and Removing Classes
Manipulating classes is a common practice for dynamic styling. You can add or remove classes to change the appearance or behavior of an element.
let element = document.getElementById("myElement");
element.classList.add("active");
element.classList.remove("hidden");
4. Creating and Appending Elements
You can dynamically create new HTML elements and append them to the DOM. This is useful for generating content on the fly.
let newElement = document.createElement("div");
newElement.textContent = "Newly created element";
document.body.appendChild(newElement);
Event Handling with JavaScript
Interactivity on webpages often revolves around user actions. JavaScript allows you to respond to these actions through event handling.
1. Adding Event Listeners
Event listeners are functions that wait for a specific event to occur on an HTML element. You can attach event listeners to elements like buttons, links, or input fields.
let button = document.getElementById("myButton");
button.addEventListener("click", function() {
// Your code here
});
2. Common Events
There’s a multitude of events you can listen for, such as click
, mouseover
, keydown
, submit
, and more. These events enable you to trigger functions when users interact with your webpage.
3. Event Object
Event listeners often receive an event object containing information about the event, such as the target element and event type. You can use this information to tailor your response.
element.addEventListener("click", function(event) {
console.log("Element clicked: " + event.target.tagName);
});
Dynamic Effects and Animations
JavaScript’s ability to manipulate HTML elements and respond to events opens up endless possibilities for creating dynamic effects and animations.
1. Sliders and Carousels
You can build interactive image sliders or content carousels that respond to user input.
2. Dropdown Menus
JavaScript allows you to create dropdown menus that appear or disappear based on user clicks.
3. Form Validation
You can use JavaScript to validate user inputs in real-time, providing feedback and preventing erroneous submissions.
4. Smooth Scrolling
JavaScript can be used to create smooth scrolling effects when users click on navigation links.
Challenges and Best Practices
While JavaScript offers incredible flexibility, it’s crucial to use it judiciously to maintain a balance between creativity and functionality:
1. Performance
Excessive use of JavaScript can slow down webpage loading times. Optimize your code and consider lazy loading for non-essential scripts.
2. Accessibility
Ensure that your dynamic effects are accessible to all users, including those with disabilities. Use ARIA roles and attributes to enhance accessibility.
3. Browser Compatibility
Be aware of browser differences and ensure that your JavaScript code works consistently across various browsers.
Conclusion
JavaScript is the driving force behind the dynamic and interactive web experiences we encounter daily. By understanding how to access, modify, and interact with HTML elements, web developers can craft captivating websites that engage and delight users. The key to success lies in striking the perfect balance between creativity and functionality, using JavaScript as a tool to enhance the user experience while maintaining performance and accessibility. As you embark on your journey as a web developer, remember that JavaScript is your ally in bringing webpages to life and making the internet a more interactive and engaging place.